See Also: Benchmarking the String and StringBuilder classes - VB.Net
String vs StringBuilder
The .Net Framework provides two different methods of dealing with strings - the String and StringBuilder classes. In this article we will look at a quick overview of the basic syntax and differences between String and StringBuilder and then run some benchmarks to compare the performance between the two using some basic operations.
The main differences between the two are that a String represents a fixed portion of memory of a specific length, and the StringBuilder uses a buffer that can dynamically grow as needed. Although one can operate on a string as if it were dynamic - i.e. s += "more stuff" - what is really happening under the covers is that .Net is creating a new string representing the concatenation of the two strings and discards the original. This can be quite costly when performing a lot of concatenations in a tight loop. On the other hand, there is more overhead involved with instantiating a StringBuilder class.
Well - it's nice to know the differences - but let's take a look at what this means in the real world. There are some differences between the two in terms of syntax.
Differences in Syntax
You instantiate a string using an assignment operator, where as a StringBuilder requires using the 'new' operator:
//Instantiate a String
String strStuff = "This is a String object.";
//Instantiate a StringBuilder
StringBuilder strbStuff
= new StringBuilder("This is a StringBuilder object.");
Concatenation is also slightly different:
//Concatenating to a String
strStuff += "Concatenating to a String object.";
//Concatenating to a StringBuilder
strbStuff.Append("Concatenating to a StringBuilder object.";
There is also a subtle but important difference when doing a search/replace operation. When using the String class, you must use an assignment operator:
//A String search/replace requires an assignment operator
strStuff = strStuff.Replace("Look for this", "Replace with this");
//But not so with the StringBuilder
strbStuff.Replace("Look for this", "Replace with this");
Differences in Performance
I first became aware of the performance differences between String and StringBuilder when I was hired to trouble-shoot a program that was experiencing performance problems and out-of-memory errors. The trouble spot involved a section of code that was doing massive amounts of search/replace and concatenation operations dealing with very large string buffers. A quick google search of 'C#' 'string' 'out of memory' 'performance' resulted in a lot of hits! Many people were experiencing similar problems with their string operations as well. The suggested solution was to use the StringBuilder class rather than the String class when doing large amounts of search/replace or especially concatenation operations.
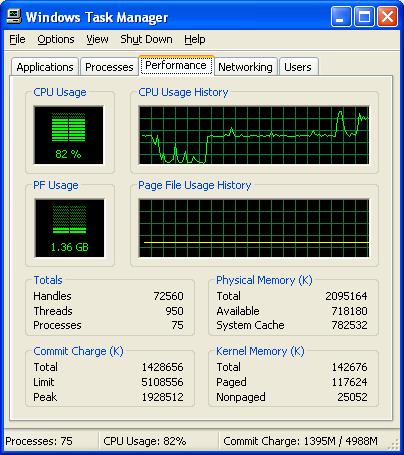
Well - to make a long story short, the StringBuilder was indeed the solution to the problem. The out of memory errors were gone, and performance improved quite substantially.
The download accompanying this article is a simple benchmark program to demonstrate the point. The program benchmarks String and StringBuilder, testing both search/replace and concatenation. Although StringBuilder noticeably out performs String in terms of search/replace - the difference is dramatic when looking at concatenation operations in a tight loop.
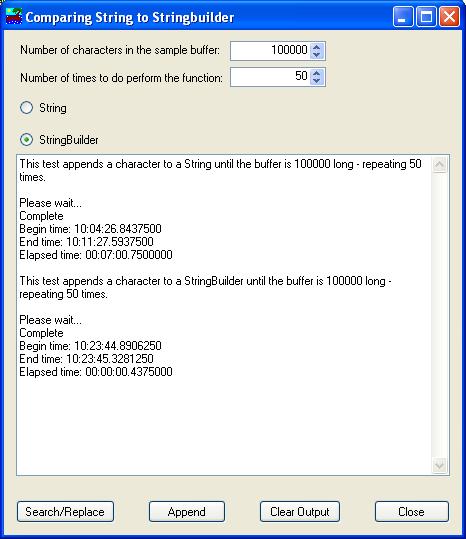
For my test, I concatenated a single character to a buffer 100,000 times and repeated these operations 50 times. This was done first for the String class and then next using StringBuilder. The results were stunning!
Using the String class took slightly over 7 minutes to complete where as the StringBuilder class was able to do the same operations in less than one second!
Conclusion
Using a String works fine for every day standard run of the mill usage, but when writing code that involves a large number of concatenation operations - you just can't beat the performance of the StringBuilder class.